DCLabel 扩展了 UILabel 的 attributedText 属性,使嵌入图像/视频内容变得简单。还包括 DCParseEngine,它提供了一个强大且可定制的解析引擎,将文本标签转换为富文本字符串。这使得简单的 HTML 和 Markdown 语法可以轻松创建和自定义富文本字符串。
将该内容转换为以下内容:
@"hello **world**! This is an _example_ of what this can do!\nNow for a markdown list:\n 1. First\n 1. Second\n 1. Third\n \
Here is [Google](http://www.google.com/). Now an image:\n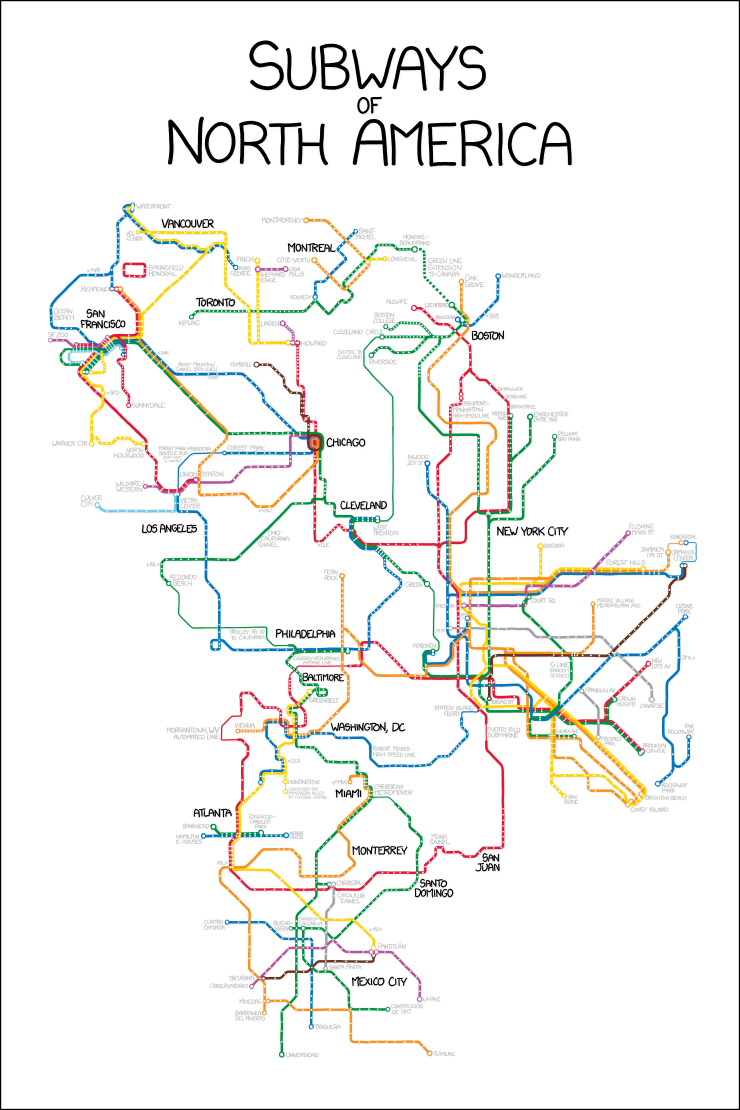\nThe possiblities are endless!"
///////////////////////////////////////////////////////////////////////
- (void)viewDidLoad
{
[super viewDidLoad];
NSString* text = @"hello **world**! This is an _example_ of what this can do!\nNow for a markdown list:\n 1. First\n 1. Second\n 1. Third\n \
Here is [Google](http://www.google.com/). Now an image:\n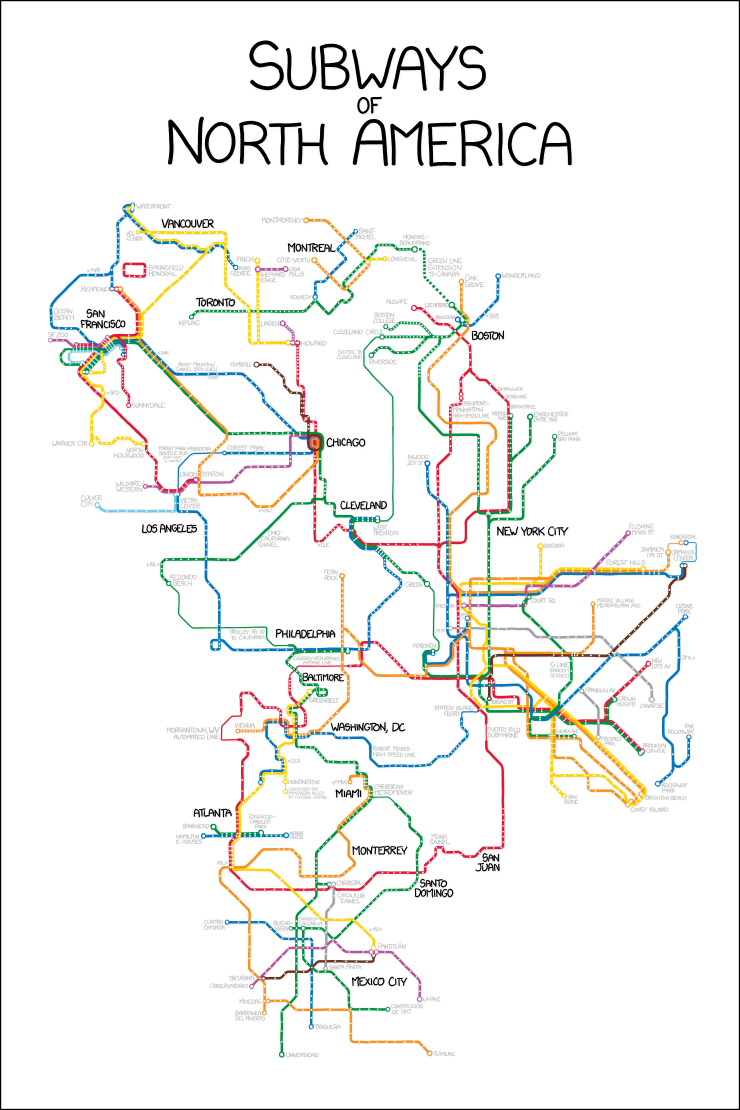\nThe possiblities are endless!";
DCParseEngine* engine = [DCParseEngine engineWithMDParser];
int pad = 6;
DCLabel* label = [[DCLabel alloc] initWithFrame:CGRectMake(pad, pad, self.view.frame.size.width-(pad*2), self.view.frame.size.height-(pad*2))];
label.delegate = self;
label.userInteractionEnabled = YES;
engine.embedWidth = label.frame.size.width;
engine.embedHeight = engine.embedWidth;
label.attributedText = [engine parse:text];
[self.view addSubview:label];
}
//DCLabel delegate methods
///////////////////////////////////////////////////////////////////////
-(UIView*)imageWillLoad:(NSString*)imgURL attributes:(NSDictionary*)attributes
{
//this can be your favorite network image viewer, does not have to be DCImageView
DCImageView* imgView = [[DCImageView alloc] init];
imgView.URL = imgURL;
[imgView start];
return imgView;
}
- (void)didSelectLink:(NSString*)link
{
NSLog(@"open a webview or a custom view of your choosing");
}
- (void)didLongPressLink:(NSString*)link frame:(CGRect)frame
{
NSLog(@"open an action of options (save,copy,open,etc)");
}
- (void)didSelectImage:(NSString*)imageURL
{
NSLog(@"open a imageViewer or a custom view of your choosing");
}
- (void)didLongPressImage:(NSString*)imageURL
{
NSLog(@"open an action of options (save,copy,open,etc)");
}
该引擎也可以进行自定义以支持你自己的标签,如下所示
DCParseEngine* engine = [[DCParseEngine alloc] init];
[engine addPattern:@"**" close:@"**" attributes:@[DC_BOLD_TEXT]];
[engine addPattern:@"__" close:@"__" attributes:@[DC_BOLD_TEXT]];
[engine addPattern:@"*" close:@"*" attributes:@[DC_ITALIC_TEXT]];
[engine addPattern:@"_" close:@"_" attributes:@[DC_ITALIC_TEXT]];
[engine addPattern:@"" block:^NSArray*(NSString* openTag,NSString* closeTag,NSString* text){
NSString* link = [closeTag substringWithRange:NSMakeRange(2, closeTag.length-3)];
return @[@{DC_IMAGE_LINK: link}];
}];
解析引擎中的?字符用作通配符。目前每个标签(包括开放和关闭标签)只支持一个通配符。
这三个属性为文本添加阴影。这三个方法与从 quartz 获取的 view.layer 的方法相同。
objective-c @property(nonatomic,strong)UIColor* textShadowColor;
设置阴影颜色。
objective-c @property(nonatomic,assign)CGSize textShadowOffset;
设置阴影的偏移量。
objective-c @property(nonatomic,assign)NSInteger textShadowBlur;
设置阴影的模糊度。
objective-c+(CGFloat)suggestedHeight:(NSAttributedString*)attributedText width:(int)width;
根据文本和宽度返回标签的建议高度。这在绘制标签并将其添加到视图层次结构之前确定 TableViewCell/ScrollView 的高度非常有用。
objective-c - (void)didSelectLink:(NSString*)link;
objective-c - (void)didLongPressLink:(NSString*)link frame:(CGRect)frame;
objective-c - (void)didSelectImage:(NSString*)imageURL;
objective-c - (void)didLongPressImage:(NSString*)imageURL;
//返回加载 imgURL 的 imageView -(UIView)imageWillLoad:(NSString)imgURL attributes:(NSDictionary*)attributes;
此框架需要至少 iOS 4 以上。推荐使用 Xcode 4。
DCLabel 受 Apache 许可证许可。