蜂鸟是一个在 Swift 中使用的抽象文本处理和模式匹配引擎(使用正则表达式)。它支持基本 Markdown 标签或突出显示用户名、链接、电子邮件等。它将字符串转换为 NSAttributedString。包括了一些常见模式,以便实现简单快捷。
特点
- 抽象且简单。创建模式既简单又灵活。
- 代码库简洁,仅有几百行代码。
示例
首先,导入框架。有关如何将框架添加到您的项目中的说明,请参阅安装指南。
import Bumblebee
这是一个简单的代码示例,但展示了强大的用例。
//add the label to the view
let label = UILabel(frame: CGRect(x: 0, y: 65, width: view.frame.size.width, height: 400))
label.numberOfLines = 0
view.addSubview(label)
let rawText = "Hello I am *red* and I am __bold__. [link here](http://vluxe.io/) Here is an image: 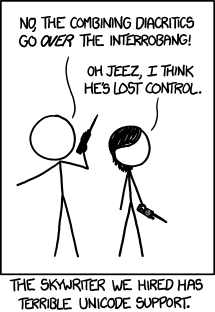. This is a second link: [Apple](https://apple.com). I like *turtles*"
parser.add(pattern: MDLinkPattern()) { (str, attributes) in
let link = attributes![MDLinkPattern.linkAttribute]!
return MatchedResponse(string: str, attributes: [NSAttributedStringKey.foregroundColor: UIColor.blue,
NSAttributedStringKey.link: URL(string: link)!])
}
parser.add(pattern: MDImagePattern()) { (str, attributes) in
let link = attributes![MDImagePattern.linkAttribute]!
let textAttachment = NSTextAttachment(data: nil, ofType: nil)
HTTP.GET(link) { (response) in
let img = UIImage(data: response.data)!
textAttachment.image = UIImage(data: response.data)
textAttachment.bounds = CGRect(x: 0, y: 0, width: img.size.width, height: img.size.height)
DispatchQueue.main.async {
label.setNeedsDisplay() //tell our label to redraw now that we have our image
}
}
return MatchedResponse(string: str, attributes: [NSAttributedStringKey.attachment: textAttachment])
}
parser.add(pattern: MDBoldPattern()) { (str, attributes) in
return MatchedResponse(string: str, attributes: [NSAttributedStringKey.font: UIFont.boldSystemFont(ofSize: 17)])
}
parser.add(pattern: MDEmphasisPattern()) { (str, attributes) in
return MatchedResponse(string: str, attributes: [NSAttributedStringKey.foregroundColor: UIColor.red])
}
parser.process(text: rawText) { (attrString) in
label.attributedText = attrString
}
看起来像这样
详情
这些模式通过正则表达式处理。创建自定义模式是通过实现 Pattern
协议来完成的。
//Matches URLs. e.g. (http://domain.com/url/etc)
public struct LinkPattern : Pattern {
public init() {} //only need to allow public initialization
public func regex() throws -> NSRegularExpression {
return try NSDataDetector(types: NSTextCheckingResult.CheckingType.link.rawValue)
}
}
//Matches typical user name patterns from social platforms like twitter. (@daltoniam, etc)
public struct UserNamePattern : Pattern {
public init() {} //only need to allow public initialization
public func regex() throws -> NSRegularExpression {
//twitter requires between 4 and 15 char for a user name, but hightlights the user name at one char...
//so I'm using {1,15} instead of {4,15}, but could be easily changed depending on requirements
return try NSRegularExpression(pattern: "(?<=\\s|^)@[a-zA-Z0-9_]{1,15}\\b", options: .caseInsensitive)
}
}
//Matches hex strings to convert them to their proper unicode version.
public struct UnicodePattern : Pattern {
public init() {} //only need to allow public initialization
public func regex() throws -> NSRegularExpression {
return try NSRegularExpression(pattern: "(?<=\\s|^)U\\+[a-zA-Z0-9]{2,6}\\b", options: .caseInsensitive)
}
//The transform method allows a pattern to do pre processing on the text before it shows up in the matched closure.
//convert the hex to its proper Unicode scalar. e.g. (U+1F602 to 😂)
public func transform(text: String) -> (text: String, attributes: [String: String]?) {
let offset = text.index(text.startIndex, offsetBy: 2)
let hex = String(text[offset..<text.endIndex])
if let i = Int(hex, radix: 16) {
let scalar = UnicodeScalar(i)
if let scalar = scalar {
return (text: String(Character(scalar)), attributes: nil)
}
}
return (text: text, attributes: nil)
}
}
//your custom pattern!
public struct MyCustomPattern : Pattern {
public init() {} //only need to allow public initialization
public func regex() throws -> NSRegularExpression {
return try NSDataDetector(types: NSTextCheckingResult.CheckingType.address.rawValue)
}
}
然后只需调用
//your custom pattern here!
parser.add(pattern: MyCustomPattern()) { (str, attributes) in
return MatchedResponse(string: str, attributes: [NSAttributedStringKey.foregroundColor: UIColor.purple])
}
更多示例请查看 bumblebee.swift
文件的底部。
安装
CocoaPods
请查阅
要在您的项目中使用Bumblebee,请在您的项目中添加以下'Podfile':
source 'https://github.com/CocoaPods/Specs.git'
platform :ios, '10.0'
use_frameworks!
pod 'Bumblebee', '~> 2.0.0'
然后执行以下操作:
pod install
Carthage
请查阅Carthage文档了解如何安装。Bumblebee框架已配置好共享方案。
Rogue
首先查看安装文档,了解如何安装Rogue。
在创建rogue文件目录下运行以下命令安装Bumblebee。
rogue add https://github.com/daltoniam/bumblebee
然后打开“libs”文件夹,将“Bumblebee.xcodeproj”添加到您的Xcode项目中。完成后,在“构建阶段”中将“Bumblebee.framework”添加到“链接二进制库”阶段。确保将“libs”文件夹添加到您的“.gitignore”文件中。
其他
简单地(通过git子模块或其他包管理器)获取框架。
将“Bumblebee.xcodeproj”添加到您的Xcode项目中。之后,在“构建阶段”中将“Bumblebee.framework”添加到“链接二进制库”阶段。
添加复制框架阶段
如果您在OSX应用程序或物理iOS设备上运行该应用程序,您需要确保您已经将包含在应用程序包中的 Bumblebee.framework
添加到应用程序中。为此,在Xcode中,通过单击蓝色项目图标,然后在侧边栏的“目标”部分下选择应用程序目标来导航到目标配置窗口。在窗口顶部的选项卡栏中,打开“构建阶段”面板。展开“链接二进制与库”组,并添加 Bumblebee.framework
。单击面板左上角的“+”按钮,并选择“新复制文件阶段”。将此新阶段重命名为“复制框架”,设置“目标”为“框架”,并添加 Bumblebee.framework
。
待办事项
- 完成单元测试
许可证
Bumblebee采用Apache v2许可证授权。